Web Application
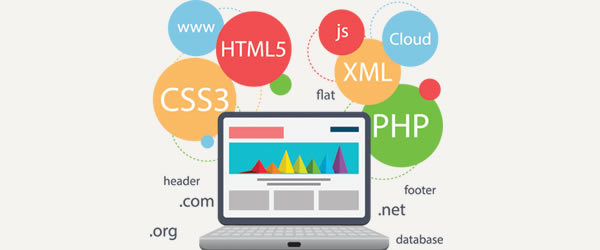
- Introduction and Background
- Historical Perspective
- What is a web application
- Web 1.0, 2.0 and 3.0
- Design Pattern
- Development Environment
- Ruby on Rails
- Rails Overview
- Build first Rails App
- A Blog App
- Rails Philosophy
- Version Control
- Git and Rails
- Create a Git Repository at Bitbucket
- Database Interactions
- Relational Databases
- Databases in Rails
- The Active Record Design Pattern
- The Blog App - Associations
- Data Validation in Web Apps
- The Ruby Programming Language
- Ruby Programming Language Background
- Classes and Inheritance
- Objects and Variables
- String, Regular Expressions and Symbols
- Expressions and Control Structures
- Collections, Blocks and Iterators
- Middleware
- What is Middleware?
- The Hypertext Transfer Protocol (HTTP)
- HTTP - Request
- HTTP - Response
- The Model-View-Controller Design Pattern
- Rails Controllers - Request Handling
- Rails Controllers - Response
- MVC Implementation in Rails
- The Blog App - Iteration 4
- Presentation and User Interface
- Introduction and Background
- HTML - Basic Syntax
- HTML - Document Structure
- HTML - Forms
- Dynamic Content, Templates and Layouts
- Cascading Style Sheets (CSS)
- Javascript & JQuery
- Ajax
- The Blog App - Iteration 5
- Others
- Web Storage
- AppCache vs. Web Storage vs. Cookie
- Cookie and Security
- What is REST?
- Browser Cache vs HTML5 Application Cache
- Web Performance
Latest Post
- Dependency injection
- Directives and Pipes
- Data binding
- HTTP Get vs. Post
- Node.js is everywhere
- MongoDB root user
- Combine JavaScript and CSS
- Inline Small JavaScript and CSS
- Minify JavaScript and CSS
- Defer Parsing of JavaScript
- Prefer Async Script Loading
- Components, Bootstrap and DOM
- What is HEAD in git?
- Show the changes in Git.
- What is AngularJS 2?
- Confidence Interval for a Population Mean
- Accuracy vs. Precision
- Sampling Distribution
- Working with the Normal Distribution
- Standardized score - Z score
- Percentile
- Evaluating the Normal Distribution
- What is Nodejs? Advantages and disadvantage?
- How do I debug Nodejs applications?
- Sync directory search using fs.readdirSync